Premium Only Content
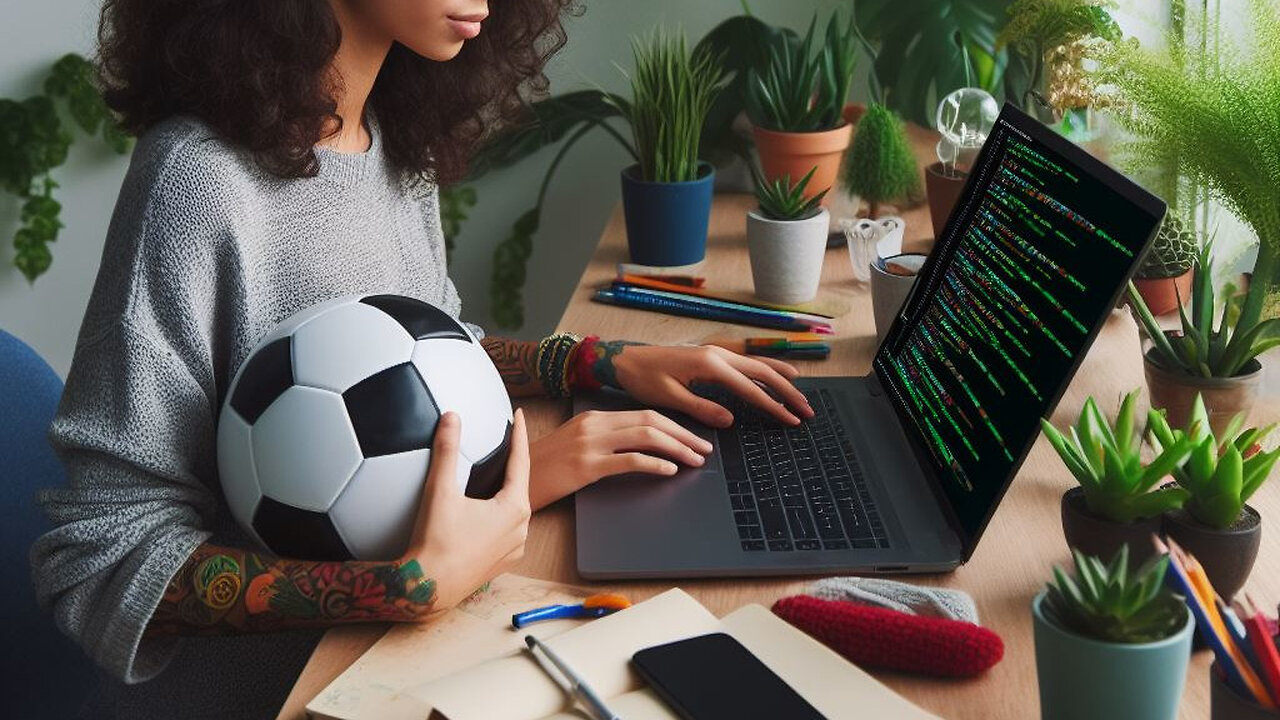
Sort Colors - Leetcode 75 - Java
Learn how to solve the Leetcode problem of id 75, whose title is Sort Colors, using the Java programming language.
https://leetcode.com/problems/sort-colors
The Data Structures and Algorithms (DSA) lesson uses a three-pointer approach to solving the question using Java.
Since the zeroes need to be placed at the left-hand side of the resulting array, we use a pointer initialized to 0 to keep track of the index for the zeroes ("Zero Pointer"). Similarly, you create another pointer initialized to length of array minus one to keep track of the index for the twos ("Two Pointer").
With a separate index pointer ("Position Pointer") to traverse the array, you look at what integer you are faced with. It will be either 0, 1, or 2.
If it's 0, swap the element at the Zero Pointer with the current position and fill in with 0 at the Zero Pointer; increment both Zero Pointer and Position Pointer.
If it's 2, swap the element at the Two Pointer with the current position, filling with 2 the value at Two Pointer; decrement the Zero Pointer.
If you find one, simply increment the Position Pointer.
The time complexity for the solution is O(n) and its space complexity is O(1).
DSA problems are sometimes asked during tech job interviews for positions such as Software Engineer, so you can use the challenge to practice that skill.
-
LIVE
GOP
11 hours agoThe 60th Presidential Inauguration Ceremony
8,150 watching -
LIVE
Donald Trump Jr.
3 hours agoFull Coverage of My Father’s Inauguration, America is Back. | TRIGGERED Ep.209
21,238 watching -
LIVE
Russell Brand
2 hours agoInauguration Day Live! – SF522
2,934 watching -
LIVE
Right Side Broadcasting Network
6 days ago🔴 LIVE: The Inauguration of Donald J. Trump as the 47th President of The United States 1/20/25
106,246 watching -
LIVE
Redacted News
3 hours agoLIVE INAUGURATION DAY COVERAGE | Trump officially takes office
8,712 watching -
1:04:17
The Dan Bongino Show
3 hours agoTrump's Historic Inauguration - LIVE FROM DC (Ep. 2404) - 01/20/2025
669K1.17K -
LIVE
LFA TV
21 hours agoLIVE: INAUGURATION OF PRESIDENT DONALD J. TRUMP
6,903 watching -
LIVE
The Quartering
21 hours agoTrump Inauguration LIVE Broadcast & Commentary With DecoyVoice, Hannah Clare & Styxhexenhammer!
4,405 watching -
1:07:17
Nerdrotic
2 hours ago $5.25 earnedReturn of the KING! - Nerdrotic Nooner 459
71K2 -
LIVE
Due Dissidence
11 hours agoLIVE: Donald Trump Inauguration - Reaction, Commentary, Reporting From DC
2,700 watching