Premium Only Content
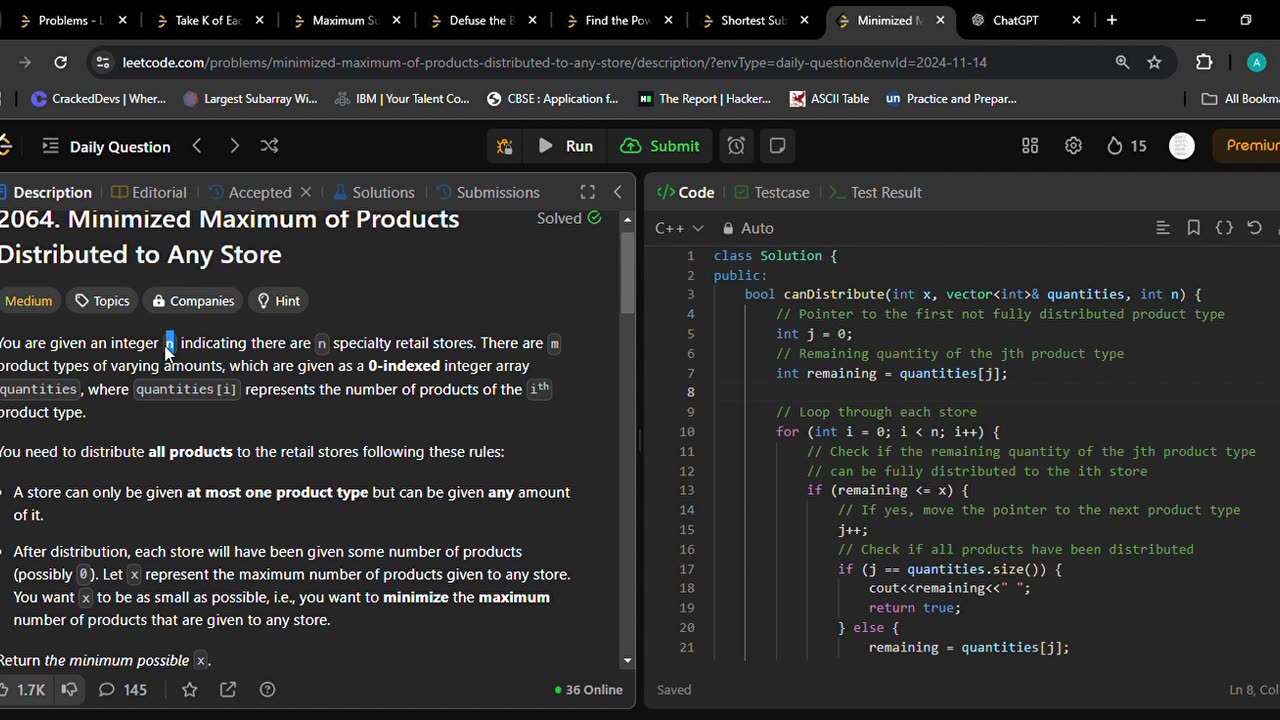
2064. Minimized Maximum of Products Distributed to Any Store
You are given an integer n indicating there are n specialty retail stores. There are m product types of varying amounts, which are given as a 0-indexed integer array quantities, where quantities[i] represents the number of products of the ith product type.
You need to distribute all products to the retail stores following these rules:
A store can only be given at most one product type but can be given any amount of it.
After distribution, each store will have been given some number of products (possibly 0). Let x represent the maximum number of products given to any store. You want x to be as small as possible, i.e., you want to minimize the maximum number of products that are given to any store.
Return the minimum possible x.
Example 1:
Input: n = 6, quantities = [11,6]
Output: 3
Explanation: One optimal way is:
- The 11 products of type 0 are distributed to the first four stores in these amounts: 2, 3, 3, 3
- The 6 products of type 1 are distributed to the other two stores in these amounts: 3, 3
The maximum number of products given to any store is max(2, 3, 3, 3, 3, 3) = 3.
Example 2:
Input: n = 7, quantities = [15,10,10]
Output: 5
Explanation: One optimal way is:
- The 15 products of type 0 are distributed to the first three stores in these amounts: 5, 5, 5
- The 10 products of type 1 are distributed to the next two stores in these amounts: 5, 5
- The 10 products of type 2 are distributed to the last two stores in these amounts: 5, 5
The maximum number of products given to any store is max(5, 5, 5, 5, 5, 5, 5) = 5.
Example 3:
Input: n = 1, quantities = [100000]
Output: 100000
Explanation: The only optimal way is:
- The 100000 products of type 0 are distributed to the only store.
The maximum number of products given to any store is max(100000) = 100000.
Constraints:
m == quantities.length
1 <= m <= n <= 105
1 <= quantities[i] <= 105
class Solution {
public:
bool canDistribute(int x, vector<int>& quantities, int n) {
// Pointer to the first not fully distributed product type
int j = 0;
// Remaining quantity of the jth product type
int remaining = quantities[j];
// Loop through each store
for (int i = 0; i < n; i++) {
// Check if the remaining quantity of the jth product type
// can be fully distributed to the ith store
if (remaining <= x) {
// If yes, move the pointer to the next product type
j++;
// Check if all products have been distributed
if (j == quantities.size()) {
cout<<remaining<<" ";
return true;
} else {
remaining = quantities[j];
}
} else {
// Distribute the maximum possible quantity (x) to the ith store
remaining -= x;
}
}
return false;
}
int minimizedMaximum(int n, vector<int>& quantities) {
// Initialize the boundaries of the binary search
int left = 0;
int right = *max_element(quantities.begin(), quantities.end());
// Perform binary search until the boundaries converge
while (left < right) {
int middle = (left + right) / 2;
if (canDistribute(middle, quantities, n)) {
// Try for a smaller maximum
right = middle;
} else {
// Increase the minimum possible maximum
left = middle + 1;
}
}
return left;
}
};
-
1:42:17
The Quartering
3 hours agoHillary Clinton FINALLY BUSTED, Nancy Pelosi MELTDOWN, Kamala Harris Admits Defeat & More
84.2K30 -
3:10:42
Barry Cunningham
8 hours agoMUST SEE: KAROLINE LEAVITT HOSTS WHITE HOUSE PRESS CONFERENCE ( AND MORE NEWS)
31.8K13 -
53:03
Sean Unpaved
4 hours agoSchlereth Unplugged: 3x Champ Talks TV, Football, & 2025 Season Expectations
14.9K -
1:03:28
Russell Brand
4 hours agoCan You Really Take an Unbiased Look at Hitler? - SF624
118K86 -
12:39
Michael Button
6 hours ago $0.84 earnedAn Entire Civilization Might Be Buried Under the Sahara
10.4K6 -
4:38
Michael Heaver
11 hours agoShifting UK Triggers Rapid REVOLUTION
7.72K1 -
58:53
The White House
3 hours agoPress Secretary Karoline Leavitt Briefs Members of the Media, July 31, 2025
24.3K38 -
5:48:07
JuicyJohns
8 hours ago $3.09 earned🟢#1 REBIRTH PLAYER 10.2+ KD🟢$500 GIVEAWAY SATURDAY!
74.4K6 -
2:08:15
IrishBreakdown
3 hours agoNotre Dame Fall Camp Practice Report
18.1K1 -
1:04:08
Timcast
4 hours agoPelosi MELTS DOWN After Trump Accuses Her Of INSIDER TADING, PELOSI ACT Moves Forward
152K93