Premium Only Content
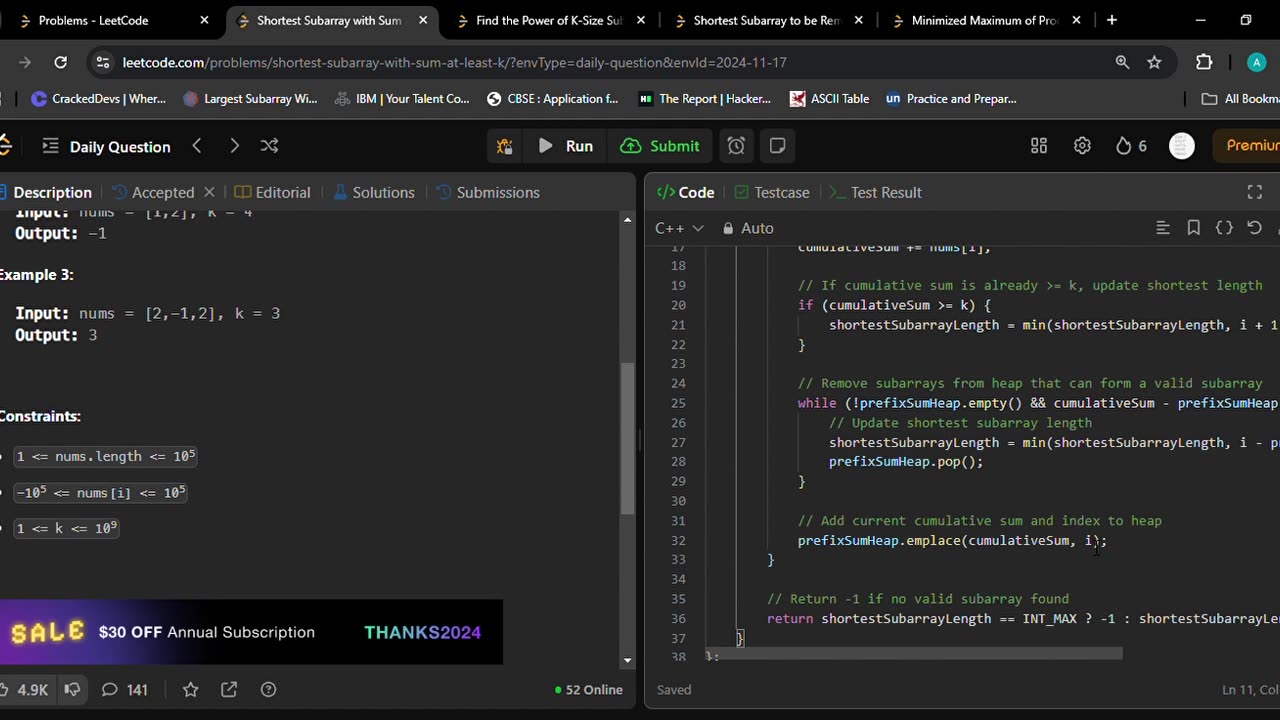
862. Shortest Subarray with Sum at Least K
Given an integer array nums and an integer k, return the length of the shortest non-empty subarray of nums with a sum of at least k. If there is no such subarray, return -1.
A subarray is a contiguous part of an array.
Example 1:
Input: nums = [1], k = 1
Output: 1
Example 2:
Input: nums = [1,2], k = 4
Output: -1
Example 3:
Input: nums = [2,-1,2], k = 3
Output: 3
Constraints:
1 <= nums.length <= 105
-105 <= nums[i] <= 105
1 <= k <= 109
class Solution {
public:
int shortestSubarray(vector<int>& nums, int k) {
int n = nums.size();
// Initialize result to the maximum possible integer value
int shortestSubarrayLength = INT_MAX;
long long cumulativeSum = 0;
// Min-heap to store cumulative sum and its corresponding index
priority_queue<pair<long long, int>, vector<pair<long long, int>>,greater<>> prefixSumHeap;
// Iterate through the array
for (int i = 0; i < n; i++) {
// Update cumulative sum
cumulativeSum += nums[i];
// If cumulative sum is already >= k, update shortest length
if (cumulativeSum >= k) {
shortestSubarrayLength = min(shortestSubarrayLength, i + 1);
}
// Remove subarrays from heap that can form a valid subarray
while (!prefixSumHeap.empty() && cumulativeSum - prefixSumHeap.top().first >= k) {
// Update shortest subarray length
shortestSubarrayLength = min(shortestSubarrayLength, i - prefixSumHeap.top().second);
prefixSumHeap.pop();
}
// Add current cumulative sum and index to heap
prefixSumHeap.emplace(cumulativeSum, i);
}
// Return -1 if no valid subarray found
return shortestSubarrayLength == INT_MAX ? -1 : shortestSubarrayLength;
}
};
-
LIVE
BSparksGaming
17 hours agoDynamic Duo! Marvel Rivals w/ Chili XDD
928 watching -
7:00:42
NellieBean
8 hours ago🔴 LIVE - trying some COD maybe Pals later
28.4K -
1:47:46
SpartakusLIVE
6 hours agoThe Master RIZZLER has entered the building, the 95% REJOICE
16.4K -
29:53
MYLUNCHBREAK CHANNEL PAGE
1 day agoOff Limits to the Public - Pt 1
72K106 -
16:03
Tundra Tactical
8 hours ago $10.44 earnedNew Age Gun Fudds
89.5K15 -
8:22
Russell Brand
13 hours agoThey want this to happen
175K364 -
2:06:43
Jewels Jones Live ®
1 day ago2025 STARTS WITH A BANG! | A Political Rendezvous - Ep. 104
96.2K35 -
4:20:41
Viss
12 hours ago🔴LIVE - PUBG Duo Dominance Viss w/ Spartakus
76.5K9 -
10:15:14
MDGgamin
16 hours ago🔴LIVE-Escape From Tarkov - 1st Saturday of 2025!!!! - #RumbleTakeover
62.8K2 -
3:54:19
SpartakusLIVE
12 hours agoPUBG Duos w/ Viss || Tactical Strategy & HARDCORE Gameplay
74.5K1