Premium Only Content
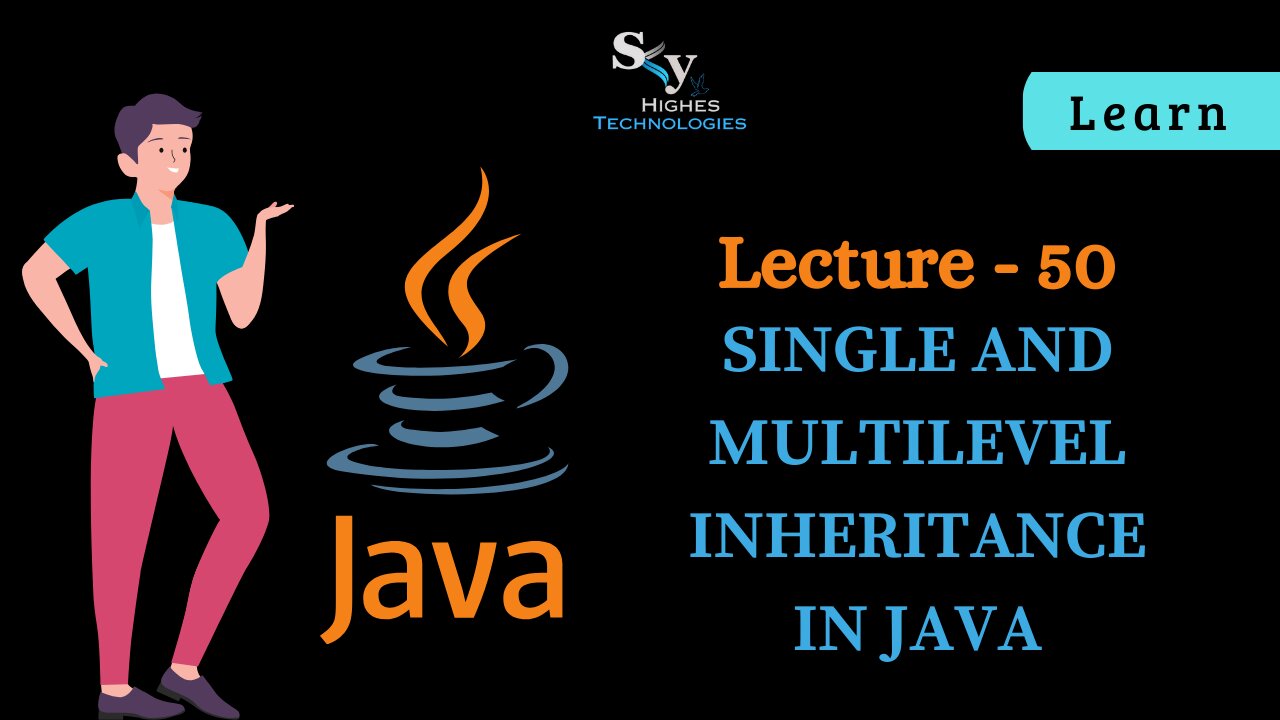
#50 Single and Multilevel inheritance in java | Skyhighes | Lecture 50
Here's a concise explanation of single and multilevel inheritance in Java, along with key differences and examples:
Single Inheritance:
Definition: A class inherits from only one direct superclass.
Structure: Parent Class -> Child Class
Example:
Java
class
Animal
{
public
void
eat()
{
System.out.println("Animal is eating");
}
}
class
Dog
extends
Animal
{
public
void
bark()
{
System.out.println("Woof!");
}
}
Use code with caution. Learn more
Multilevel Inheritance:
Definition: A class inherits from a class that is itself a subclass of another class, creating a chain of inheritance.
Structure: Parent Class -> Intermediate Class -> Child Class
Example:
Java
class Animal {
// ...
}
class Mammal extends Animal {
// ...
}
class Dog extends Mammal {
// ...
}
Use code with caution. Learn more
Key Differences:
Feature Single Inheritance Multilevel Inheritance
Inheritance path Direct single-level inheritance Chain of inheritance
Complexity Simpler to manage Can become more complex with deep hierarchies
Code reusability Limited to one level of hierarchy Allows reuse across multiple levels
Best Practices:
Use inheritance judiciously: Excessive inheritance can create complex and tightly coupled code.
Favor composition over inheritance when possible: Composition (using objects as members) can provide more flexible relationships.
Prefer shallow hierarchies: Avoid deep multilevel inheritance for better maintainability.
Consider interfaces for multiple inheritance-like behavior: Java supports multiple inheritance of interfaces, allowing classes to implement multiple sets of behaviors.
Remember: Choose the type of inheritance that best suits your code's structure and requirements, balancing reusability with maintainability.
-
28:09
Michael Franzese
17 hours agoThese Athletes Are STEALING Gold Medals From Women | Deep Dive
94.7K54 -
6:04:01
TheItalianCEO
10 hours agoGTA V RP First Time EVER (help)
86.5K4 -
15:22
Russell Brand
2 days agoSO IT BEGINS.....
300K361 -
5:41
Chef Donny
2 days agoPan Frying Dumplings With Thanksgiving Leftovers | Tasty Tailgating Ep. 12
68.2K1 -
6:50:37
Scottish Viking Gaming
10 hours agoSTALKER 2 | Sunday Funday | The Zone makes my Brain Fuzzy
48.2K2 -
3:57:16
LumpyPotatoX2
11 hours agoNew Fortnite Season - #RumbleGaming
56.8K3 -
15:06
Forrest Galante
1 day agoShooting Invasive Flying Carp with a Bow
169K52 -
6:39:16
Akademiks
1 day agoThis is How Drake Comes Back!! Kendrick First Week Sales in. Diddy, Durk, YSL Update.
333K63 -
10:29
TimcastIRL
2 days agoJoe Rogan SLAMS Joe Biden For ESCALATING The War In Ukraine
182K251 -
14:21
Scammer Payback
26 days agoHacked Scammer Laptop to find their location
99.6K64