Premium Only Content
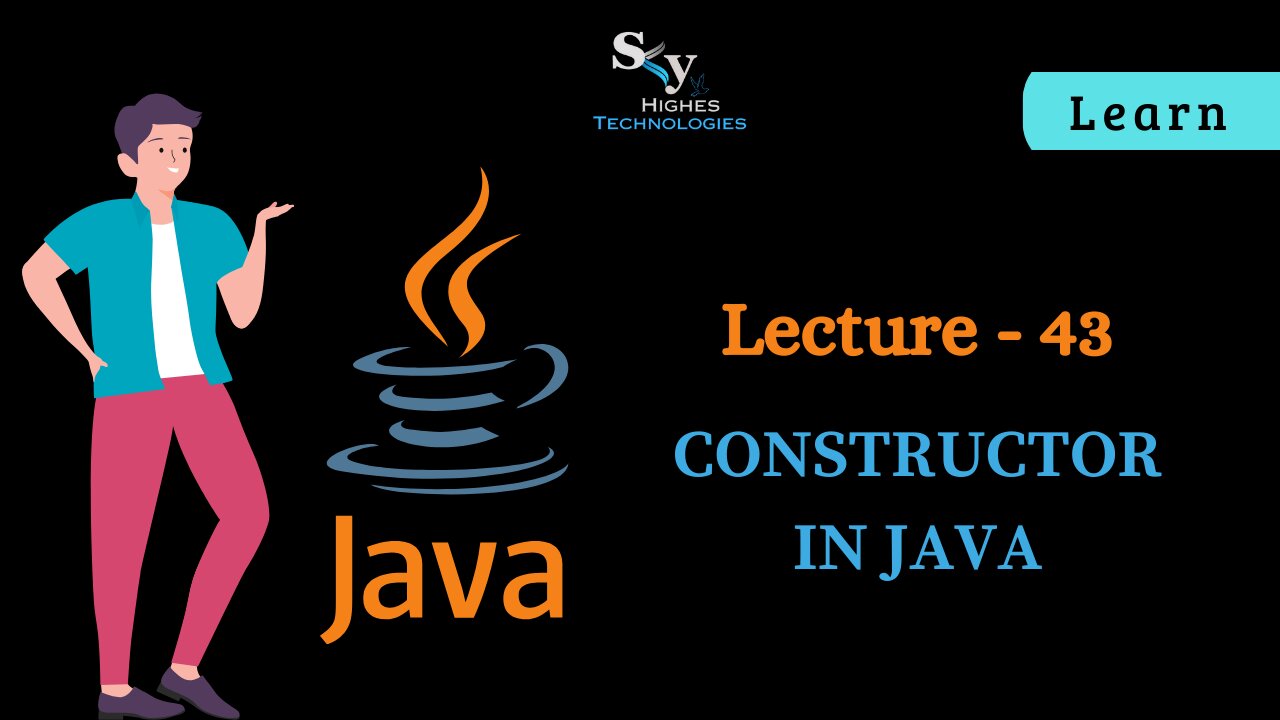
#43 Constructor in JAVA | Skyhighes | Lecture 43
Here's a comprehensive explanation of constructors in Java:
What are constructors?
Special methods: They are responsible for initializing the state of an object when it's created.
Same name as the class: They have the same name as the class they belong to, distinguishing them from regular methods.
No return type: They don't have a return type, not even void.
Purpose of constructors:
Initialization: To set initial values for the object's instance variables.
Resource allocation: To acquire resources like database connections or file handles that the object will need during its lifetime.
Preparation: To perform any other setup tasks necessary for the object to function properly.
Types of constructors:
Default constructor: A constructor with no arguments. If you don't explicitly define any constructors, Java provides a default constructor that does nothing.
Parameterized constructor: A constructor that takes one or more arguments to initialize the object's state with specific values.
How constructors are called:
Using the new keyword: Constructors are called using the new keyword followed by the class name and any required arguments:
Java
ClassName objectName = new ClassName(arguments);
Use code with caution. Learn more
Example:
Java
class Person {
private String name;
private int age;
// Default constructor
public
Person()
{
this.name = "";
this.age = 0;
}
// Parameterized constructor
public
Person(String name, int age)
{
this.name = name;
this.age = age;
}
}
Use code with caution. Learn more
Key points:
Every class has at least one constructor, even if you don't explicitly define it (the default constructor).
Constructors can be overloaded (multiple constructors with different parameter lists).
The this keyword is often used within constructors to refer to the current object being created.
Constructors cannot be inherited, but they can be called using super() from a subclass.
Understanding constructors is essential for creating and working with objects in Java. By effectively using constructors, you can ensure that objects are properly initialized and ready for use in your code.
-
1:14:28
vivafrei
2 hours agoSCOTUS Rules Against Trans "Treatments" as Cory Booker Cries! Guardian Goes Silent! Butler PA & MORE
50.5K23 -
LIVE
StoneMountain64
3 hours agoBlack Ops 7 NO WallRunning, No Blackout 2 & Warzone Bugs Addressed
388 watching -
LIVE
Pop Culture Crisis
2 hours agoAI SEDUCES Married Man, Chappell Roan CRIES Over Haters, Pedro Pascal Gives Women THE ICK | Ep. 860
397 watching -
1:54:18
The Quartering
3 hours agoTrump Moves Bombers Into Position, Man Leaves Feminist GF For AI, Trump SHUTS DOWN Woke Hotline
97.3K24 -
35:39
Michael Franzese
2 hours agoFormer Mob Boss Reveals What It Really Takes To Be a REAL Leader
7.73K3 -
LIVE
The HotSeat
1 hour agoThe Military Does NOT Care About An Influencer's Opinion! Stay In Your LANE!
759 watching -
LIVE
The Nunn Report - w/ Dan Nunn
1 hour ago[Ep 692] Tucker’s TED Talk | Too-Late Powell | Leftists Now Backing Iran!!!
298 watching -
1:10:00
HotZone
4 hours ago $3.71 earned🔴 LIVE: Tehran Evacuates: Israel Crushes Iran’s Last Missile Strike and Russia Threatens Trump
21.8K13 -
1:38:23
TheDozenPodcast
3 hours agoTommy Robinson Prison Exclusive | Solitary Confinement & LIES Exposed
2.87K7 -
1:39:20
Russell Brand
4 hours ago9/11 – Still Just a Conspiracy Theory in 2025? - SF600
150K27