Premium Only Content
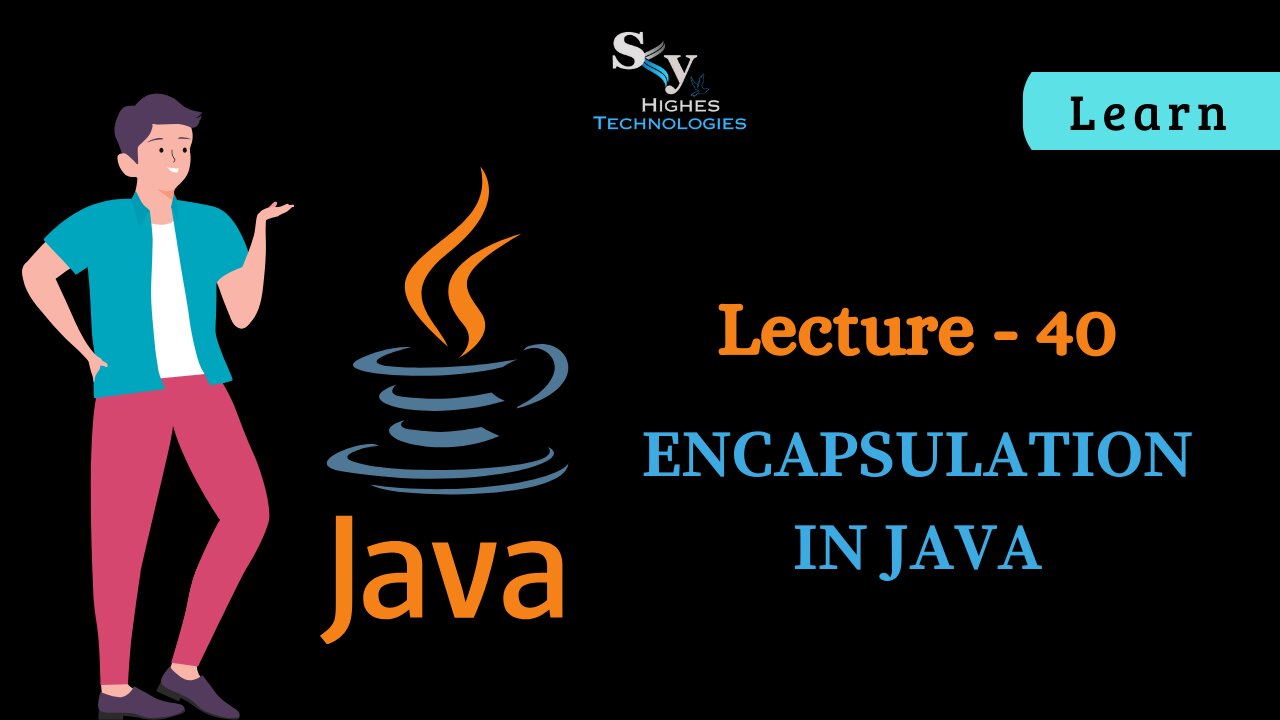
#40 Encapsulation in JAVA | Skyhighes | Lecture 40
Here's a comprehensive explanation of encapsulation in Java:
What is encapsulation?
Fundamental OOP concept: It involves binding data (variables) and the code that operates on that data (methods) together within a single unit, called a class.
Data hiding: It's primarily about protecting the internal state of an object from direct external access, ensuring data integrity and consistency.
Key mechanisms for implementing encapsulation:
Access modifiers:
private: Restricts access to members within the same class, enforcing data hiding.
public: Allows access from anywhere, typically used for methods that provide the interface to interact with the object.
protected: Allows access within the class and its subclasses, promoting code reusability.
Getter and setter methods:
Getters (accessors): Public methods that provide controlled access to private data members.
Setters (mutators): Public methods that allow controlled modification of private data members, often with data validation or other logic.
Example:
Java
class BankAccount {
private int balance; // Private data member
public void deposit(int amount) {
if (amount > 0) {
balance += amount;
} else {
System.out.println("Invalid deposit amount.");
}
}
public int getBalance() {
return balance;
}
}
Use code with caution. Learn more
Benefits of encapsulation:
Data integrity: Prevents accidental or unauthorized modification of data, ensuring its consistency.
Modularity: Promotes code organization and reusability by isolating classes and their responsibilities.
Flexibility: Allows changes to internal implementation without affecting external code that interacts with the class.
Maintainability: Makes code easier to understand, test, and manage due to clear boundaries and controlled access.
Security: Can enhance security by restricting access to sensitive data or operations.
Best practices:
Make data members private by default.
Provide public getters and setters only when necessary.
Validate data in setter methods to ensure integrity.
Design classes with clear responsibilities and well-defined interfaces.
Avoid exposing internal implementation details unnecessarily.
-
5:43:44
Scammer Payback
2 days agoCalling Scammers Live
215K28 -
18:38
VSiNLive
2 days agoProfessional Gambler Steve Fezzik LOVES this UNDERVALUED Point Spread!
150K20 -
LIVE
Right Side Broadcasting Network
10 days agoLIVE REPLAY: President Donald J. Trump Keynotes TPUSA’s AmFest 2024 Conference - 12/22/24
2,511 watching -
4:31
CoachTY
1 day ago $28.72 earnedCOINBASE AND DESCI !!!!
193K13 -
10:02
MichaelBisping
1 day agoBISPING: "Was FURY ROBBED?!" | Oleksandr Usyk vs Tyson Fury 2 INSTANT REACTION
113K16 -
8:08
Guns & Gadgets 2nd Amendment News
2 days ago16 States Join Forces To Sue Firearm Manufacturers Out of Business - 1st Target = GLOCK
132K93 -
10:17
Dermatologist Dr. Dustin Portela
2 days ago $19.59 earnedOlay Cleansing Melts: Dermatologist's Honest Review
164K17 -
1:02:20
Trumpet Daily
2 days ago $52.45 earnedObama’s Fake World Comes Crashing Down - Trumpet Daily | Dec. 20, 2024
121K86 -
6:29
BIG NEM
1 day agoCultivating God Mode: Ancient Taoist NoFap Practices
86.2K22 -
30:53
Uncommon Sense In Current Times
2 days ago $12.38 earned"Pardon or Peril? How Biden’s Clemency Actions Could Backfire"
96.1K10