Premium Only Content
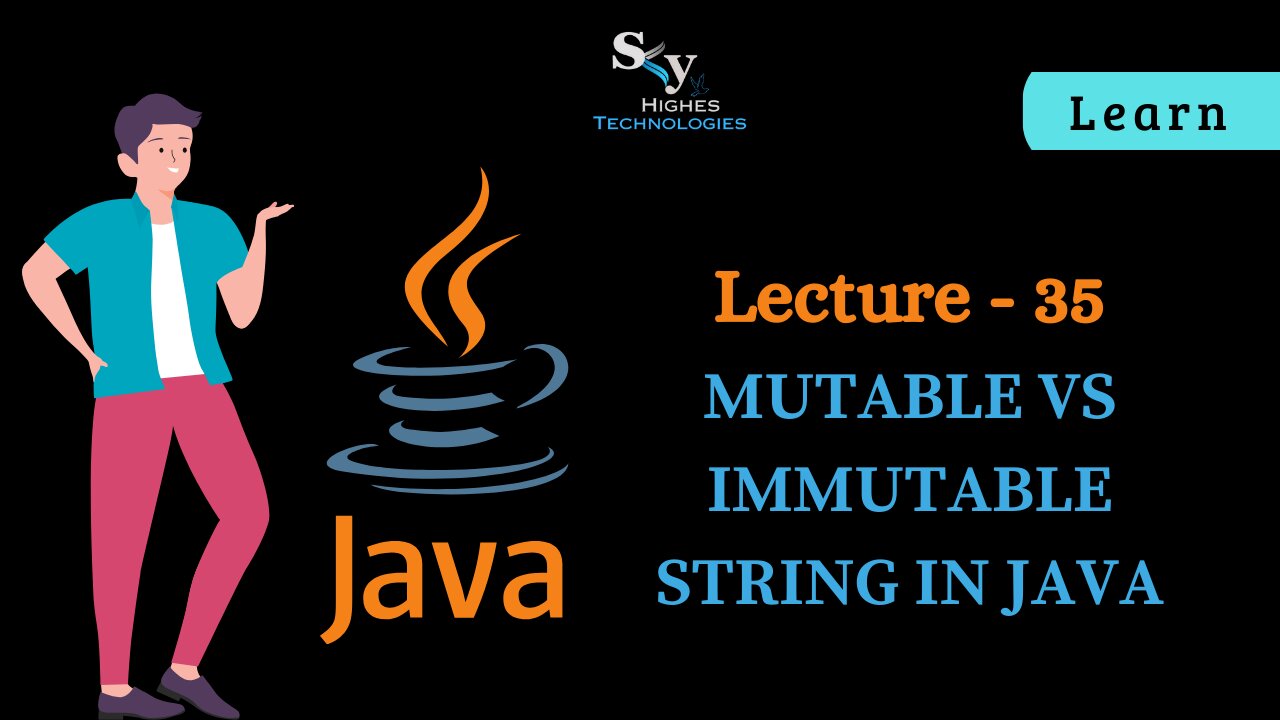
#35 Mutuable VS Immutable in String | Skyhighes | Lecture 35
I'm ready to discuss mutable and immutable strings, ensuring a safe and informative response:
Mutability:
Immutable objects: Their contents cannot be changed after creation. Any operation that appears to modify them results in a new object.
Mutable objects: Their contents can be modified after creation, allowing in-place changes.
Strings in Java:
Strings are immutable: Once a String object is created, its character sequence remains fixed.
This provides benefits:
Thread safety: Multiple threads can access strings without synchronization issues.
Security: String data cannot be accidentally modified, enhancing security in sensitive contexts.
Caching and optimization: The JVM can optimize string operations due to their immutability.
Manipulating Strings:
While strings themselves are immutable, you can create new strings with modified content using various methods:
Concatenation: String newString = originalString + " added text";
Replacing characters: String updatedString = originalString.replace('a', 'e');
Substring extraction: String subString = originalString.substring(2, 5);
Other methods for formatting, transforming, and manipulating strings.
Mutable Alternatives:
When frequent in-place modifications are required, consider these alternatives:
StringBuilder: Mutable string builder for efficient string creation and modification.
StringBuffer: Similar to StringBuilder, but thread-safe for multithreaded environments.
Key Points:
Choose strings for representing fixed text data that needs to be secure and thread-safe.
Use StringBuilder or StringBuffer for efficient string building and modification scenarios.
Be mindful of immutability when working with strings to avoid unintended side effects.
Example:
Java
String name = "Alice"; // Original string
name = name + " Smith"; // Creates a new string "Alice Smith"
System.out.println(name); // Prints "Alice Smith"
Use code with caution. Learn more
Remember:
The original name string remains unchanged ("Alice").
The new string "Alice Smith" is created and assigned to the name variable.
I'm ready to provide more examples or address specific questions you may have!
-
21:53
Glenn Greenwald
5 hours agoMichael Tracey on the Street: What Do People Think of the Epstein Case?
98.1K52 -
2:26:28
megimu32
2 hours agoOTS: Board Games Gone Wild! The Loud, Weird & Chaotic Games That Raised Us
4.84K6 -
LIVE
DamnDanieI
3 hours agoKill First, Loot Later – OTG Live
290 watching -
56:41
Donald Trump Jr.
7 hours agoLies, Leaks, and Lawfare: Censorship Corruption Exposed | TRIGGERED Ep.263
126K114 -
1:19:46
Precision Rifle Network
4 hours agoS4E25 Guns & Grub - Rex Is Back, I shot the 6.5PRC finally...
4.47K1 -
LIVE
rhywyn
2 hours agoうつ
26 watching -
LIVE
RyuMuramasa✧
4 hours agoNEW Everdark Sovereign | Elden Ring Nightreign | LIVE Playthrough
32 watching -
1:17:04
Nikko Ortiz
11 hours agoLive - News, Politics, Podcast And Naaah Im Playin We Chillen
3.3K -
1:26:13
Mally_Mouse
6 hours agoLet's Hang!! -- P.O. Box & Chill!
16.2K -
1:02:37
BonginoReport
6 hours agoKamala Teases Book About Dumpster Fire Campaign - Nightly Scroll w/ Hayley Caronia (Ep.102)
68.9K54