Premium Only Content
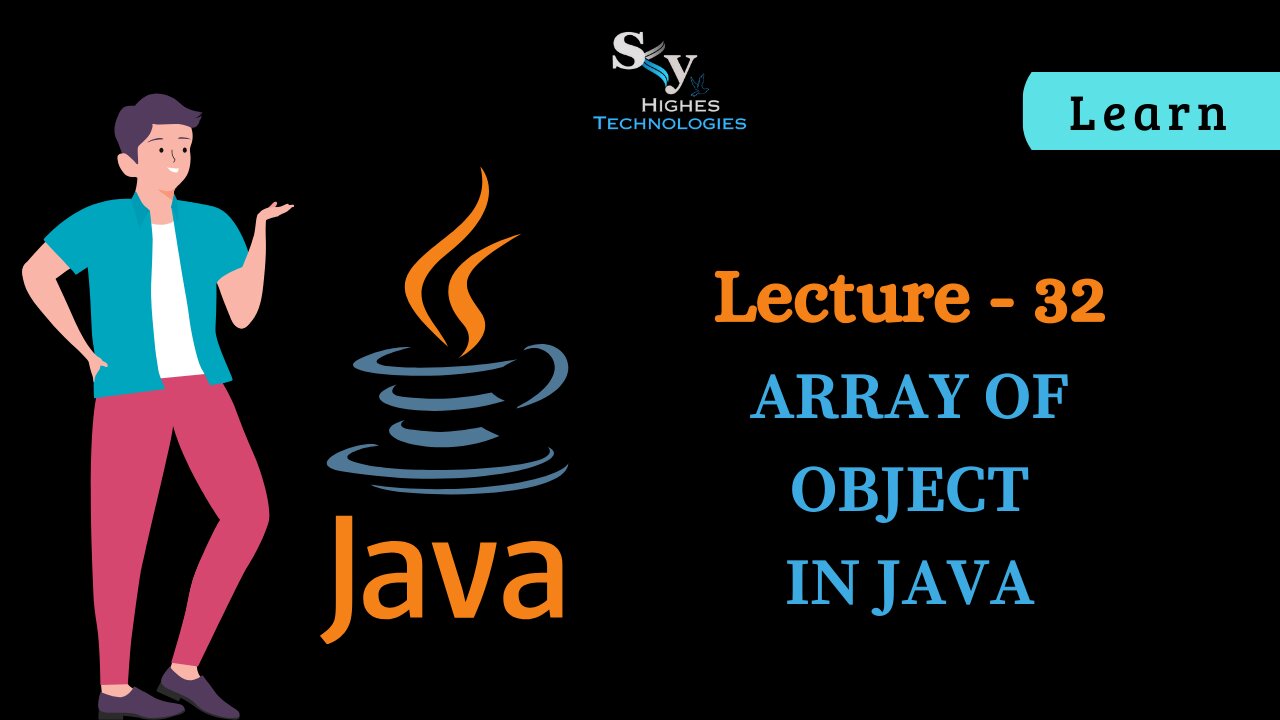
#32 Array of Object in JAVA | Skyhiges | Lecture 32
Here's a comprehensive explanation of arrays of objects in Java:
Storing Objects in Arrays:
While arrays typically store primitive data types, Java also allows you to store objects.
This enables you to manage collections of objects with similar properties and behaviors, using convenient array features.
Creating an Array of Objects:
Declare the array using the class name:
Java
Employee[] employees = new Employee[5]; // Declares an array that can hold 5 Employee objects
Use code with caution. Learn more
Instantiate each object individually:
Java
employees[0] = new Employee("John", "Doe", 25);
employees[1] = new Employee("Jane", "Smith", 30);
Use code with caution. Learn more
Accessing Object Members:
Use the array index to access the object, then the dot notation to access its members:
Java
System.out.println(employees[0].getName()); // Prints "John"
employees[1].setAge(35); // Updates the age of the second employee
Use code with caution. Learn more
Key Points:
Arrays store references to objects, not the objects themselves.
Each object in the array is independent, with its own state and behavior.
Modifying an object in the array doesn't affect other objects in the array.
Benefits of Using Arrays of Objects:
Organize similar objects: Group objects of the same type for efficient management.
Iterate and process collections: Use loops to apply operations to all objects in the array.
Pass as arguments and return values: Pass entire arrays to methods or return them as results.
Create multidimensional arrays: Store matrices or grids of objects for complex data structures.
Example:
Java
class Employee {
private String name;
private String role;
private int age;
// Constructor and getters/setters omitted for brevity
}
// Create an array of Employee objects
Employee[] team = new Employee[3];
team[0] = new Employee("Alice", "Developer", 32);
team[1] = new Employee("Bob", "Manager", 45);
team[2] = new Employee("Charlie", "Designer", 28);
// Print the names of all employees
for (Employee emp : team) {
System.out.println(emp.getName());
}
Use code with caution. Learn more
Remember:
Choose arrays of objects when you need to manage a collection of similar objects with a fixed size.
For dynamic collections with more flexibility, consider using lists or other Java Collections Framework classes.
-
2:12:58
Robert Gouveia
5 hours agoSenator's Wife EXPOSED! Special Counsel ATTACKS; AP News BLOWN OUT
61.5K39 -
55:07
LFA TV
1 day agoDefending the Indefensible | TRUMPET DAILY 2.25.25 7PM
26.1K13 -
6:09:26
Barry Cunningham
11 hours agoTRUMP DAILY BRIEFING - WATCH WHITE HOUSE PRESS CONFERENCE LIVE! EXECUTIVE ORDERS AND MORE!
70.2K45 -
1:46:37
Game On!
6 hours ago $3.16 earnedPUMP THE BRAKES! Checking Today's Sports Betting Lines!
36.7K2 -
1:27:21
Redacted News
6 hours agoBREAKING! SOMETHING BIG IS HAPPENING AT THE CIA AND FBI RIGHT NOW, AS KASH PATEL CLEANS HOUSE
183K215 -
1:08:28
In The Litter Box w/ Jewels & Catturd
1 day agoCrenshaw Threatens Tucker | In the Litter Box w/ Jewels & Catturd – Ep. 749 – 2/25/2025
107K49 -
44:57
Standpoint with Gabe Groisman
1 day agoWill Byron Donalds Run for Florida Governor? With Congressman Byron Donalds
54.9K9 -
1:06:25
Savanah Hernandez
5 hours agoEXPOSED: FBI destroys evidence as NSA’s LGBTQ sex chats get leaked?!
77.1K28 -
1:59:58
Revenge of the Cis
7 hours agoEpisode 1452: Hindsight
65K10 -
1:20:35
Awaken With JP
9 hours agoCrenshaw Threatens to Kill Tucker and Other Wild Happenings - LIES Ep 80
129K73