Premium Only Content
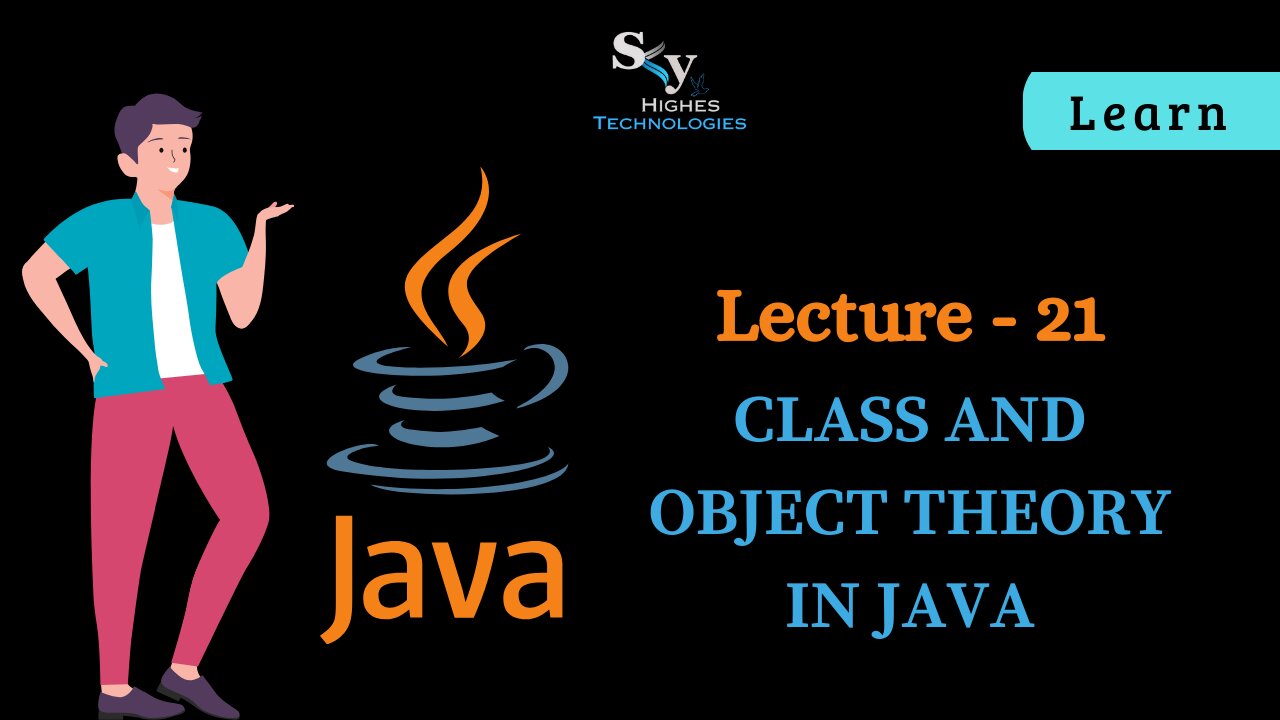
#21 Class and Object Theory in JAVA | Skyhighes | Lecture 21
Classes and Objects: Foundations of OOP
Object-Oriented Programming (OOP): A programming paradigm that revolves around "objects" as fundamental building blocks. It models real-world entities and their relationships, promoting code reusability, maintainability, and modularity.
Classes: Blueprints or templates that define the characteristics (attributes) and behaviors (methods) of objects. They specify what objects of that class will look like and what they can do.
Objects: Instances of classes. They are concrete entities with specific state (values of their attributes) and the ability to perform actions (methods).
Key Concepts:
Class Declaration:
Use the class keyword followed by the class name.
Enclose attributes (variables) and methods (functions) within curly braces.
Java
public class Dog {
String breed;
int age;
void bark() {
System.out.println("Woof!");
}
}
Use code with caution. Learn more
Object Creation (Instantiation):
Use the new keyword followed by the class name and a constructor call.
Java
Dog myDog = new Dog();
Use code with caution. Learn more
Attributes (Member Variables):
Represent the data associated with an object.
Define them within the class using various data types (e.g., int, String, boolean).
Methods (Member Functions):
Define the actions that objects can perform.
Contain code blocks that operate on the object's attributes and other data.
Accessing and Modifying Object State:
Use the dot (.) operator to access attributes and methods of an object.
Java
myDog.breed = "Labrador";
myDog.bark(); // Output: "Woof!"
Use code with caution. Learn more
Understanding the Relationship:
A class is like a recipe for creating objects.
Each object is a unique instance of the class, with its own set of attribute values.
Objects interact with each other by calling each other's methods.
Benefits of OOP:
Encapsulation: Bundling data and behavior within objects, protecting data integrity.
Inheritance: Creating new classes (subclasses) that inherit properties and behaviors from existing classes (superclasses), promoting code reusability.
Polymorphism: Objects of different classes responding to the same method call in different ways, allowing for flexible and adaptable code.
Remember: Classes and objects are fundamental to OOP and essential for structuring Java programs effectively. Understanding their relationship and properties is crucial for building well-organized and maintainable software.
-
LIVE
Robert Gouveia
2 hours agoSupreme Court Unloads! Straight Woman WINS! 2A Victory! Spitting Lib in MORE Trouble!
1,640 watching -
LIVE
LFA TV
21 hours agoLFA TV ALL DAY STREAM - THURSDAY 6/5/25
1,311 watching -
LIVE
Badlands Media
19 hours agoQuite Frankly Ep. 9: "The New Ouija Board & Musk CRASHES OUT" ft Rev. Bill Bean 6/6/25
329 watching -
1:10:28
Kim Iversen
2 hours agoMusk Drops BOMB: "Trump Is In The Epstein Files"
54K117 -
1:15:09
Ark of Grace Ministries
5 hours agoProphetic Warning: These Eruptions Are All Connected…
22.7K7 -
1:21:25
Dr. Drew
6 hours agoEpstein Accuser: Was Diddy A CIA Blackmail Asset? w/ Anouska de Georgiou & Emilie Hagen (LIVE From Sean "Diddy" Combs Trial in Manhattan) – Ask Dr. Drew
12.3K7 -
1:57:41
Redacted News
3 hours agoTucker Carlson WARNS Trump that Neo-cons are trying to END his presidency by going to war with Iran
100K34 -
53:32
Kimberly Guilfoyle
4 hours agoThe Fight for Accountability, Live with Martha Byrne & Daniel Turner | Ep227
30.9K5 -
1:28:55
The Officer Tatum
3 hours agoLIVE: Elon Musk DROPS BOMB On Donald Trump + MORE | EP 118
38.4K24 -
1:46:26
Roseanne Barr
4 hours agoDon't Let Commies Split Maga W/ James Lindsay | The Roseanne Barr Podcast #102
53K20