Premium Only Content
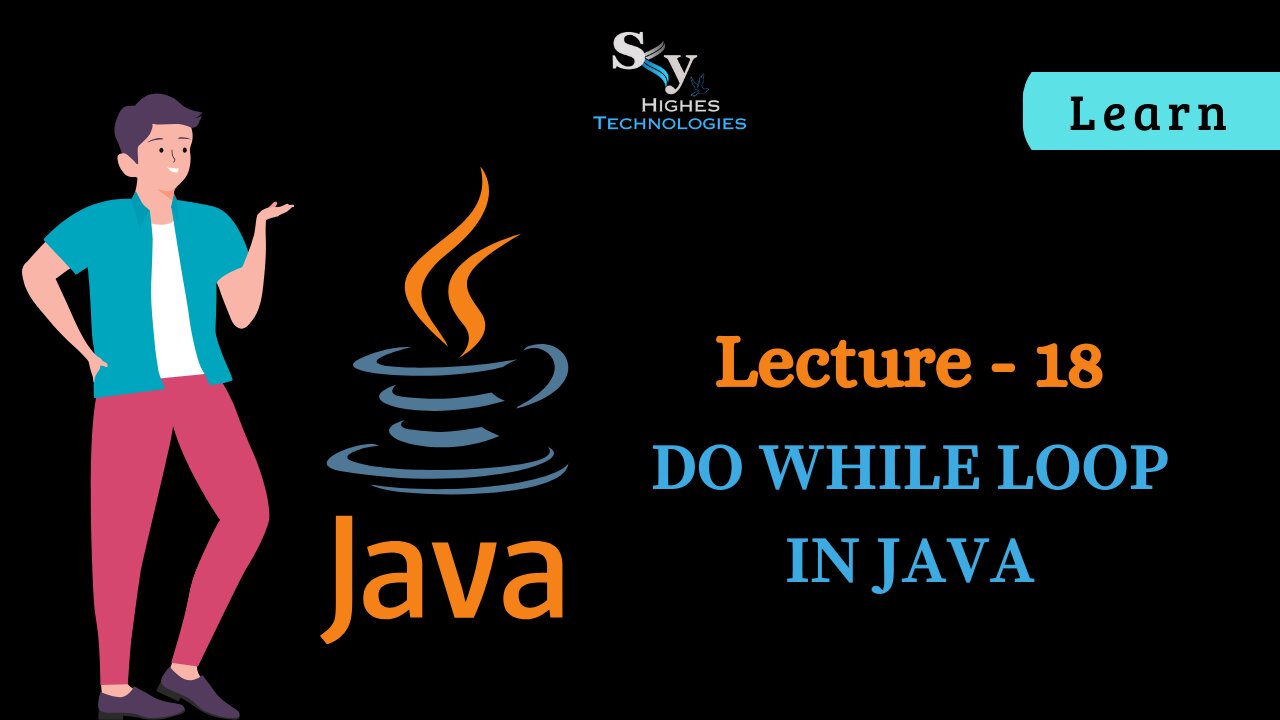
#18 Do while Loop In JAVA | Skyhighes | Lecture 18
Do-While Loops in Java: The "At Least Once" Guarantee
Imagine you have a task that needs to be done at least once, even if the condition for repetition isn't initially met. That's where the do-while loop steps in, a close cousin of the while loop with a unique twist.
Here's how it works:
Action First, Questions Later: The code block within the loop executes before the condition is checked for the first time.
Condition Check: After the initial execution, the condition is evaluated.
Loop or Exit: If the condition is true, the loop body executes again. This cycle continues until the condition becomes false.
Guaranteed Execution: This ensures that the code block runs at least once, even if the condition is initially false.
Syntax:
Java
do {
// Code to be executed at least once
} while (condition);
Use code with caution. Learn more
Example:
Java
int choice = 0;
do {
System.out.println("Enter a number (1-5): ");
choice = input.nextInt(); // Assume input is a Scanner object
} while (choice < 1 || choice > 5); // Keep asking until a valid choice is made
Use code with caution. Learn more
Key Points:
Guaranteed Execution: The code block always runs at least once, making it ideal for tasks that require initialization or input before checking conditions.
Condition Check: The condition is still crucial, as it determines whether the loop continues after the initial execution.
Syntax Difference: The do keyword comes before the code block, and the while statement with the condition comes after.
Common Use Cases:
Prompting for user input until a valid value is entered
Implementing menus that should always display at least once
Reading data from a file until the end is reached (ensuring at least one read attempt)
Handling game logic that requires an initial action before checking game state
Remember: Do-while loops offer flexibility by ensuring a minimum execution. However, use them judiciously to avoid unintended consequences. When in doubt, the standard while loop is often a safer choice for general repetition tasks.
-
LIVE
NEWSMAX
6 months agoNEWSMAX2 LIVE | Real News for Real People
2,499 watching -
LIVE
Joe Donuts Live
1 hour ago🟢LIVE with the BOYS : Tales From The Gulag
111 watching -
LIVE
Boxin
2 hours agoToday We start Fable 2!
759 watching -
25:23
The Connect: With Johnny Mitchell
1 day ago $1.12 earnedWhy They're Lying To You About The LA Riots, ICE Raids, & The Future Surveillance State
4.73K18 -
2:38:44
The Charlie Kirk Show
11 hours agoLIVE: US Bombs Iran - Trump Speaking Soon
442K722 -
58:03
FRENCHY4185
1 hour agoSPLITGATE 2 : PLAYING W/ FOLLOWERS
7 -
LIVE
bogebop
1 hour agoNo Man's Sky - LIVE -
102 watching -
LIVE
Lofi Girl
2 years agoSynthwave Radio 🌌 - beats to chill/game to
143 watching -
58:11
Right Side Broadcasting Network
11 hours agoLIVE: President Trump Addresses the Nation Following U.S. Attack on Iran's Nuclear Sites - 6/21/25
338K243 -
8:00:06
GamersErr0r
10 hours ago $0.09 earnedBig Mac Daddy Bronze King Slay | Marvel Rivals | Rank Terrorist
4.32K1