Premium Only Content
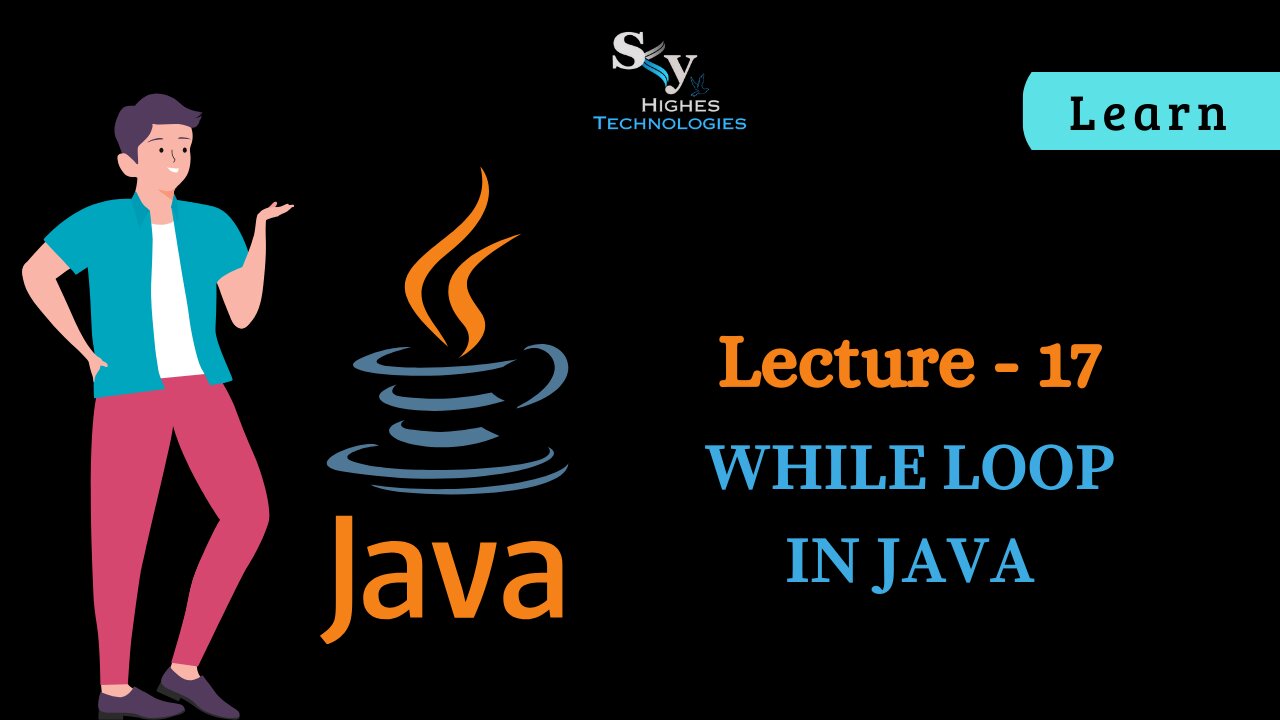
#17 While Loop in JAVA | Skyhighes | Lecture 17
While Loops in Java: The Persistent Adventurers
Think of a while loop as a determined explorer, venturing into unknown territory until a specific condition is met. It's willing to repeat tasks as long as necessary to achieve its goal.
Here's how it works:
Initial Check: The loop begins by evaluating a boolean condition.
Loop Body Execution: If the condition is true, the code block within the loop executes.
Condition Re-evaluation: After each execution of the code block, the condition is checked again.
Repetition or Termination: If the condition remains true, the loop body executes again. This cycle continues until the condition becomes false.
Moving On: Once the condition is false, the loop terminates, and the program moves on to the next instruction.
Syntax:
Java
while (condition) {
// Code to be repeated
}
Use code with caution. Learn more
Example:
Java
int i = 1;
while (i <= 5) {
System.out.println("Counting: " + i);
i++; // Increment i to avoid an infinite loop
}
Use code with caution. Learn more
Key Points:
Condition is Key: The loop hinges on the condition's truth value. Ensure it can eventually become false to prevent infinite loops.
Code Block: Contains the code to be repeated as long as the condition holds true.
Infinite Loop Risk: If the condition never becomes false, the loop will run indefinitely, potentially crashing your program.
Break Statements: Use break within the loop to exit prematurely if needed.
Common Use Cases:
Reading user input until a specific value is entered
Processing data until the end of a file is reached
Implementing game loops that run until the game ends
Generating random numbers until a certain condition is met
Implementing algorithms that require repeated steps
Remember: While loops are powerful tools for repetition, but use them wisely. Always ensure the condition can eventually become false, and consider using break statements when appropriate to avoid unexpected behavior. By mastering while loops, you'll empower your Java code to tackle repetitive tasks with determination and precision!
-
LIVE
Pepkilla
3 hours agoZeeVerse and Pixel ~ Crypto
100 watching -
8:42
Degenerate Jay
5 hours ago $4.74 earnedWow! Silent Hill 1 Remake Is Really Happening!
26.7K3 -
LIVE
Shield_PR_Gaming
2 hours agoCarXStreet with racing wheel! Should Rumble Rants Non-Taxable Donations! Are they already!?
54 watching -
LIVE
GritsGG
1 day agoSpecialist Every Game! 👑 2587+ Ws
331 watching -
1:27:21
Game On!
13 hours ago $5.50 earnedGame 4 NBA Finals is One We Have NEVER Seen Before!
35.3K3 -
23:16
Stephen Gardner
20 hours ago🚨BREAKING: DOGE EXPOSES NEW money laundering as Israel does MASS PsyOp on Iran!
68.2K155 -
14:59
T-SPLY
20 hours agoKilmar Is Facing Trafficking Charges / Lawyer Demands His Release!
51.8K29 -
1:40:18
Cowboy Logic
16 hours agoCowboy Logic - 06/14/25: Full Show
8.31K -
1:02:05
Trumpet Daily
1 day ago $3.76 earnedThe Rising Lion - Trumpet Daily | June 13, 2025
10.5K19 -
2:47
Memology 101
18 hours ago $0.86 earnedWe were THIS close🤏 to a CATASTROPHE of APOCALYPTIC proportions...
9.71K9