Premium Only Content
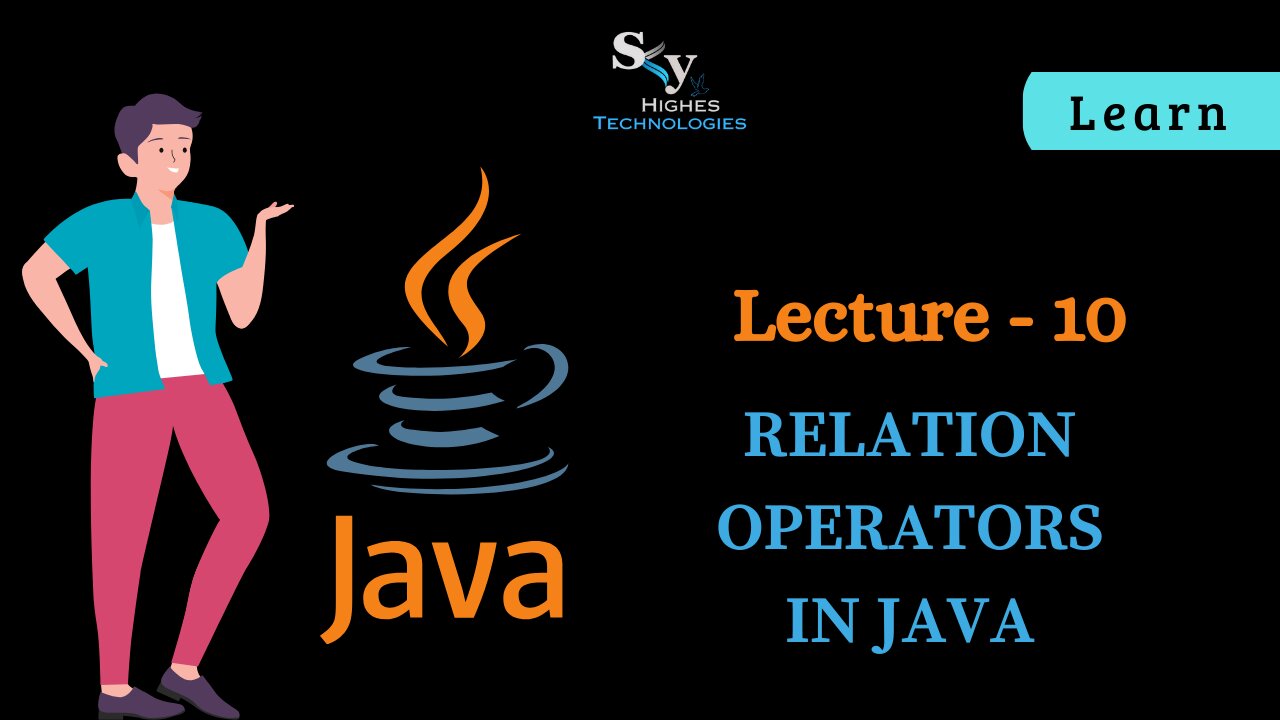
#10 Relational operators in JAVA | Skyhighes | Lecture 10
Here's a breakdown of relational operators in Java, ready to make comparisons in your code:
Relational operators are like those trusty scales in your code, weighing values and determining their relationships. They ask questions like, "Is this number bigger? Is this string different? Are these values equal?"
Here are the key players:
Equal to (==): Checks if two values are identical.
Not equal to (!=): Checks if two values are different.
Greater than (>): Checks if the left operand is larger than the right operand.
Less than (<): Checks if the left operand is smaller than the right operand.
Greater than or equal to (>=): Checks if the left operand is larger than or equal to the right operand.
Less than or equal to (<=): Checks if the left operand is smaller than or equal to the right operand.
How to use them:
Place the operator between the values or variables you want to compare.
The result of a relational operation is always a boolean value: true or false.
Examples:
Java
int age = 25;
boolean isAdult = age >= 18; // true
String name = "Alice";
boolean isBob = name == "Bob"; // false
double price = 9.99;
boolean isExpensive = price > 10.0; // false
Use code with caution. Learn more
Common use cases:
Making decisions in conditional statements (like if and else)
Filtering data in loops
Validating user input
Sorting data
Key points:
Relational operators work with various data types, including numbers, strings, and booleans.
They are essential for building logic and decision-making into your Java programs.
Use parentheses to clarify the order of operations when multiple relational operators are involved.
Remember: Relational operators are like the judges of your code, making logical comparisons to guide your program's flow. Master them, and you'll unlock a whole new level of decision-making power in your Java creations!
-
58:10
Kimberly Guilfoyle
3 hours agoAmerica is Back & The Future is Bright: A Year in Review | Ep. 183
15.2K14 -
vivafrei
8 hours agoEp. 242: Barnes is BACK AGAIN! Trump, Fani, J6, RFK, Chip Roy, USS Liberty AND MORE! Viva & Barnes
52.8K21 -
LIVE
Dr Disrespect
6 hours ago🔴LIVE - DR DISRESPECT - MARVEL RIVALS - GOLD VANGUARD
3,666 watching -
1:15:00
Awaken With JP
5 hours agoMerry Christmas NOT Happy Holidays! Special - LIES Ep 71
75.8K81 -
1:42:21
The Quartering
7 hours agoTrump To INVADE Mexico, Take Back Panama Canal Too! NYC Human Torch & Matt Gaetz Report Drops!
61.6K43 -
2:23:15
Nerdrotic
6 hours ago $8.26 earnedA Very Merry Christmas | FNT Square Up - Nerdrotic Nooner 453
42.9K4 -
1:14:05
Tucker Carlson
6 hours ago“I’ll Win With or Without You,” Teamsters Union President Reveals Kamala Harris’s Famous Last Words
122K269 -
1:58:31
The Dilley Show
6 hours ago $27.24 earnedTrump Conquering Western Hemisphere? w/Author Brenden Dilley 12/23/2024
109K27 -
1:09:59
Geeks + Gamers
7 hours agoSonic 3 DESTROYS Mufasa And Disney, Naughty Dog Actress SLAMS Gamers Over Intergalactic
70.5K17 -
51:59
The Dan Bongino Show
8 hours agoDemocrat Donor Admits The Scary Truth (Ep. 2393) - 12/23/2024
739K2.28K