Premium Only Content
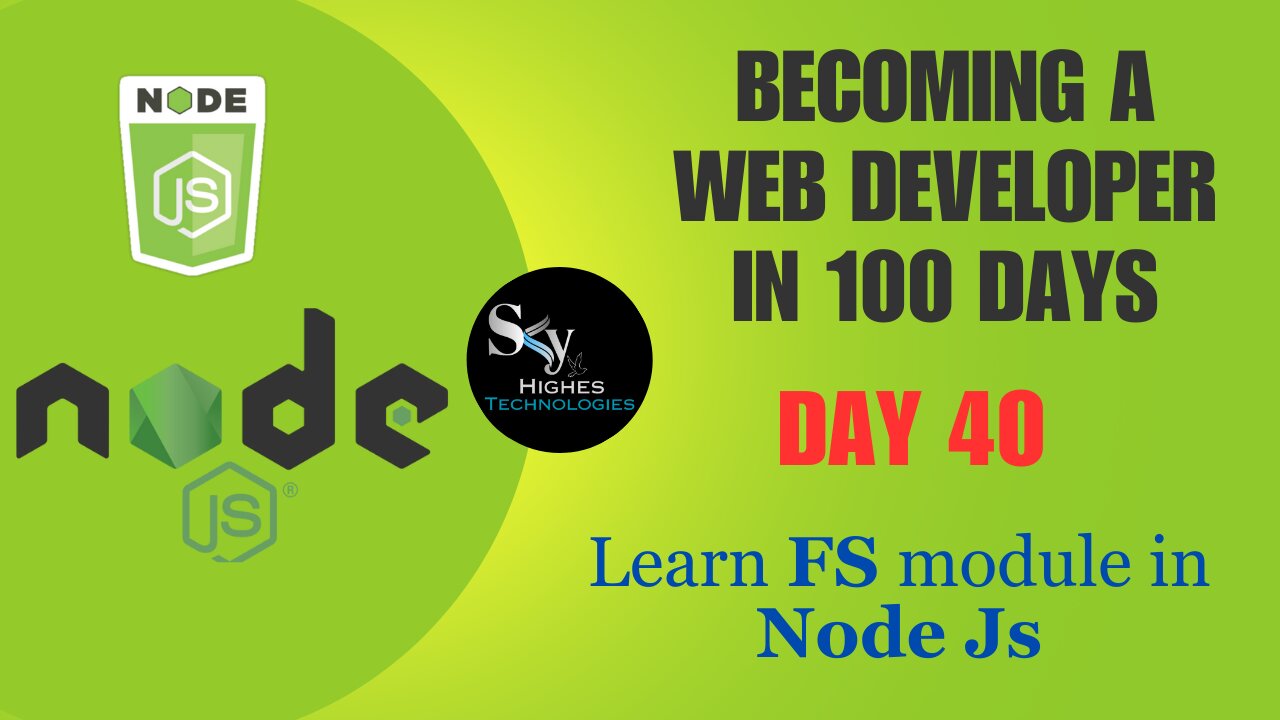
Learn FS Modules in Node.js | Render HTML File in Web Server | Day 40 |web development course 2023
I'm ready to guide you through the essential aspects of the File System (fs) module in Node.js:
Understanding the fs Module:
It's a built-in module that provides a wide range of functionalities for interacting with the file system, allowing you to read, write, delete, and manage files and directories on your server.
Accessing the fs Module:
To use it in your Node.js project, import it using the require() function:
JavaScript
const fs = require('fs');
Use code with caution. Learn more
Key Methods and Operations:
Reading Files:
fs.readFile(filename, [options], callback): Reads the entire contents of a file asynchronously.
fs.readFileSync(filename, [options]): Synchronous version (blocks execution until reading is complete).
Writing Files:
fs.writeFile(filename, data, [options], callback): Writes data to a file, creating it if it doesn't exist.
fs.writeFileSync(filename, data, [options]): Synchronous version.
Appending to Files:
fs.appendFile(filename, data, [options], callback): Appends data to the end of an existing file.
Deleting Files:
fs.unlink(path, callback): Deletes a file.
Creating Directories:
fs.mkdir(path, [options], callback): Creates a new directory.
Listing Files and Directories:
fs.readdir(path, [options], callback): Reads the contents of a directory (lists files and subdirectories).
Checking File Existence:
fs.exists(path, callback): Checks if a file or directory exists.
Getting File Information:
fs.stat(path, callback): Gets detailed information about a file, such as size, permissions, and modification time.
-
LIVE
Vigilant News Network
13 hours agoEXPOSED: Secret Government Plot to Deploy Aerosolized ‘Vaccines’ Using Drones | Media Blackout
1,667 watching -
1:13:49
Josh Pate's College Football Show
10 hours ago $7.22 earnedSemifinal Predictions: OhioSt v Texas | Notre Dame v PennSt | Playoff Cinderella | Alabama’s Future
67.7K2 -
27:56
The Why Files
1 day agoThe Seventh Experiment: Lacerta Reveals the Truth of our Creation
126K67 -
45:53
hickok45
21 hours agoSunday Shoot-a-Round # 262
49.4K19 -
4:52:32
Rotella Games
1 day agoGrand Theft America - GTA IV | Day 1
88.8K7 -
8:16:19
Joe Donuts Gaming
21 hours ago🟢Fortnite Live : Chill Vibes Lounge!
101K9 -
38:43
Standpoint with Gabe Groisman
17 hours agoEp. 63. Terror Strikes the Nova Music Festival. Ofir Amir
165K53 -
36:04
Forrest Galante
1 day agoPrivate Tour of an Indian Billionaire’s Secret Wildlife Rescue Center
130K19 -
9:37
Space Ice
1 day agoMorbius Is The Perfect Movie, Everyone Just Lied To You - Best Movie Ever
114K32 -
17:09
Guns & Gadgets 2nd Amendment News
1 day agoWhy I Left The USCCA
99.4K48