Premium Only Content
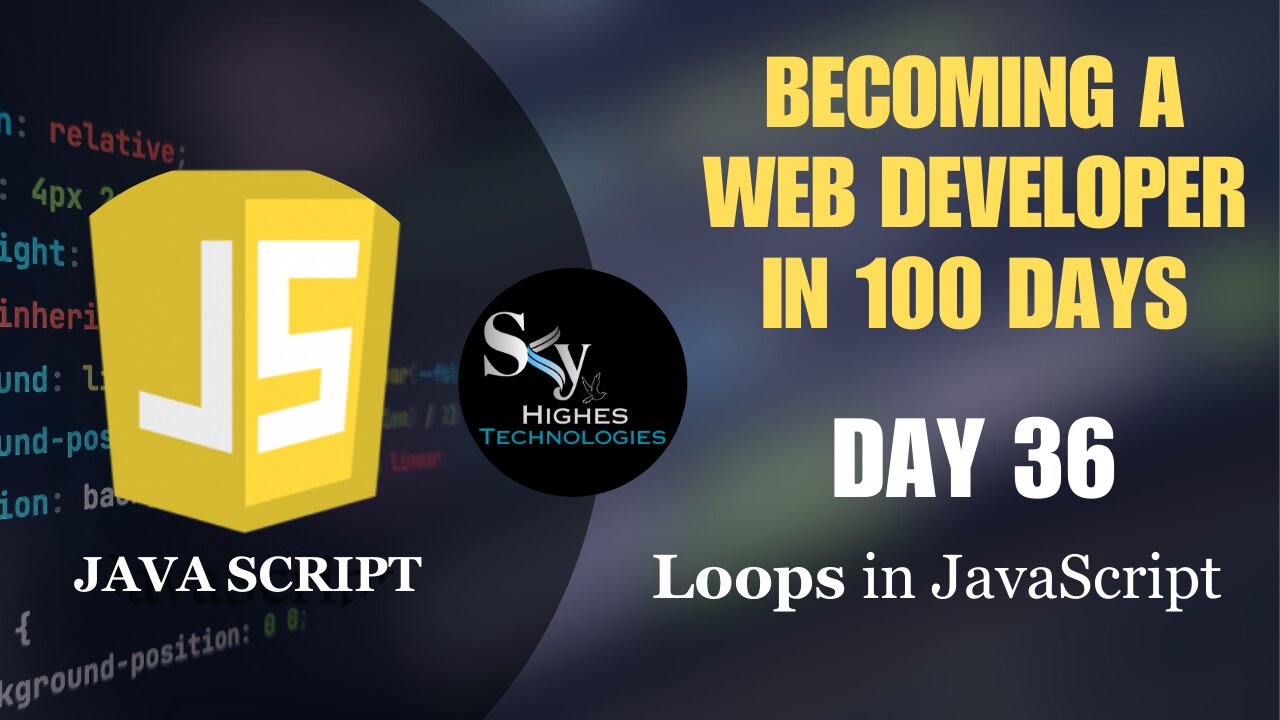
Loops in JavaScript | Day 36 | For loop And While Loop | Web development course 2023
View other drafts
1
Loops in JavaScript
Loops are control flow statements that allow you to execute a block of code repeatedly until a specific condition is met. They are essential for automating tasks and processing large amounts of data. There are three primary loop types in JavaScript:
1. For loop:
The for loop iterates a block of code a specified number of times. It uses the following syntax:
JavaScript
for (initial_expression; condition; update_expression) {
// code to be executed
}
Use code with caution. Learn more
initial_expression: Initializes a counter variable.
condition: Determines whether the loop continues.
update_expression: Updates the counter variable after each iteration.
2. While loop:
The while loop iterates a block of code as long as a condition is true. It uses the following syntax:
JavaScript
while (condition) {
// code to be executed
}
Use code with caution. Learn more
condition: Determines whether the loop continues.
3. Do...while loop:
The do...while loop is similar to the while loop, but it executes the code block at least once before checking the condition. It uses the following syntax:
JavaScript
do {
// code to be executed
} while (condition);
Use code with caution. Learn more
condition: Determines whether the loop continues.
Here are some examples of each loop type:
1. For loop:
JavaScript
for (let i = 0; i < 5; i++) {
console.log(i); // Outputs: 0, 1, 2, 3, 4
}
Use code with caution. Learn more
2. While loop:
JavaScript
let count = 0;
while (count < 5) {
console.log(count); // Outputs: 0, 1, 2, 3, 4
count++;
}
Use code with caution. Learn more
3. Do...while loop:
JavaScript
let input;
do {
input = prompt("Enter a number:");
} while (isNaN(input)); // Ensures valid input
console.log("You entered:", input);
Use code with caution. Learn more
In addition to these basic loop types, JavaScript offers several other loop variations and control flow statements for more complex situations. These include:
for...in loop: Iterates over the properties of an object.
for...of loop: Iterates over the values of an iterable object.
break statement: Exits the current loop.
continue statement: Skips the current iteration of the loop and continues to the next.
-
LIVE
Russell Brand
1 hour agoBudget Cuts, Fires, and the Failures of Leadership – SF523
9,795 watching -
LIVE
The Charlie Kirk Show
1 hour agoAmerica's New Golden Age: Day 2 | Kelly, Kane | 1.21.2025
16,597 watching -
UPCOMING
The Dan Bongino Show
5 hours ago[LIVE AT 3 ET] HE'S BACK! (Ep. 2405) - 01/21/2025
316K328 -
LIVE
TheAlecLaceShow
1 hour agoDonald Trump Becomes President | Interviews & Reaction From Inauguration Day | The Alec Lace Show
86 watching -
35:10
Grant Stinchfield
20 hours agoDr. Drew Reveals The Video YouTube Refuses to Let You See! We Un-Censor it!
2.57K4 -
LIVE
The Dana Show with Dana Loesch
1 hour agoTRUMP BEGINS SECOND TERM | The Dana Show LIVE On Rumble!
1,119 watching -
50:06
The Rubin Report
2 hours agoThese Unexpected Executive Orders Signed by Trump Are Game-Changers with Co-Host Russell Brand
80.3K57 -
1:59:33
Steven Crowder
4 hours agoEXPLAINED: Donald Trump’s Day One Orders & Elon’s Fake Nazi Hand Gesture
471K206 -
3:48:59
Right Side Broadcasting Network
13 hours agoLIVE REPLAY: President Trump Attends National Prayer Service in Washington, D.C. - 1/21/25
135K97 -
1:47:32
Graham Allen
5 hours agoBack From The BRINK! Trump Erases The Biden Disaster On Day ONE!!
98.3K76