Premium Only Content
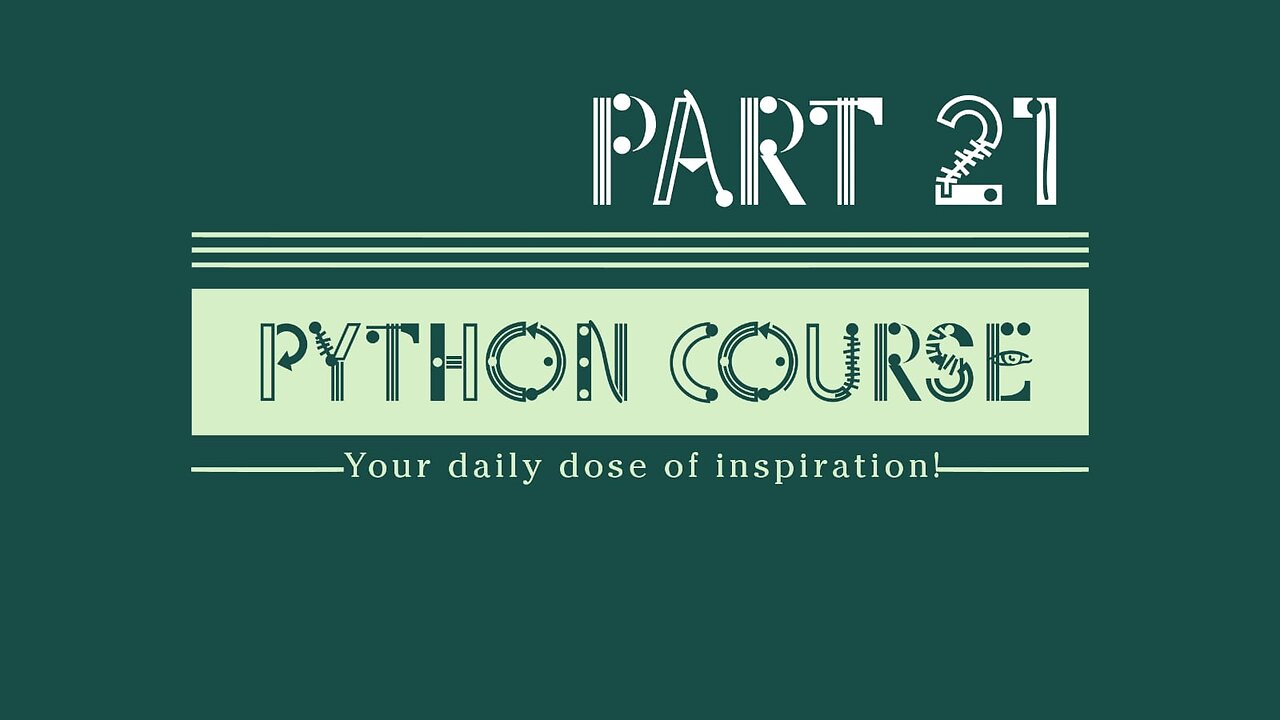
Exception Handling in Python |Section 2|Celestial Warrior
1
00:00:01,129 --> 00:00:06,899
Hi again! Let's have one more lecture
about errors in Python and this
3
00:00:06,899 --> 00:00:11,429
particular lecture will be about
handling errors. Handling errors can be
5
00:00:11,429 --> 00:00:16,590
an advanced concept to digest for now
because you're still going through the
7
00:00:16,590 --> 00:00:22,199
basics of Python and you haven't seen
any real application yet, but later
9
00:00:22,199 --> 00:00:27,990
will use error handling while we are
building real-world applications so
11
00:00:27,990 --> 00:00:32,399
you're going to understand error
handling better later on. For now let's
13
00:00:32,399 --> 00:00:35,670
just focus on the syntax and try to
understand as much as you can
15
00:00:35,670 --> 00:00:42,899
I'll go and do an example here which I
mentioned earlier. Let's say we have a
17
00:00:42,899 --> 00:00:54,090
function that does division between two
given numbers like that. Return a divided
19
00:00:54,090 --> 00:00:59,719
by b and then you call the function here.
Divide 1, 2. In this case the
21
00:01:05,640 --> 00:01:10,619
function will try to divide 1 by 2.
And let me print out the output so that
23
00:01:10,619 --> 00:01:20,159
we can see it on the terminal. Go to the
terminal and execute the script. So you
25
00:01:20,159 --> 00:01:28,460
get you get 0.5. Now that's good.
However if the user enters let's say
27
00:01:28,460 --> 00:01:35,790
zero there like that, execute and your
program will crash so it will show an
29
00:01:35,790 --> 00:01:41,939
error. Now this one here is an example of
a longer error message that I mentioned
31
00:01:41,939 --> 00:01:45,750
previously in the previous videos that
you might have more than one, more
33
00:01:45,750 --> 00:01:54,869
than one error in your traceback, so for
instance this says line 4 at this line
35
00:01:54,869 --> 00:02:01,259
here. So that line is where Python
captured the error at first
37
00:02:01,259 --> 00:02:06,360
so basically Python was trying to
execute this line but it couldn't do it,
39
00:02:06,360 --> 00:02:10,709
it didn't manage to do it because this
line was trying to get the information
41
00:02:10,709 --> 00:02:15,460
from this function
and this function was trying to do this
43
00:02:15,460 --> 00:02:22,280
mathematical operation using zero after
the division so that is not possible and
45
00:02:22,280 --> 00:02:27,950
Python is pointing that out here.
So that's the main error in line two
47
00:02:27,950 --> 00:02:32,510
in this expression so that's where you
should focus, however when you ask
49
00:02:32,510 --> 00:02:36,590
questions you should include the entire
error message in your question
51
00:02:36,590 --> 00:02:43,550
as I mentioned previously.
So back to handling errors now. What I'm
53
00:02:43,550 --> 00:02:49,850
trying to say here is that you know
you have other functions here and other
55
00:02:49,850 --> 00:02:57,320
lines of code that you want to execute,
that you want Python to execute, but if
57
00:02:57,320 --> 00:03:04,720
the user passed this zero number here
the other lines would not be executed
59
00:03:04,720 --> 00:03:09,680
so your program would crash and you
don't want that and the way to avoid
61
00:03:09,680 --> 00:03:17,720
that is by using try and accept so
basically you try to capture the
63
00:03:17,720 --> 00:03:24,170
exception, to handle the exception.
The way to do that is quite easy.
65
00:03:24,170 --> 00:03:33,860
You say try and return a/b, so this has to be
indented like that so try is indented
67
00:03:33,860 --> 00:03:40,160
because it's under the function
definition. And then return is indented
69
00:03:40,160 --> 00:03:49,190
because it's under the try expression.
Then you have except, execute so this
71
00:03:49,190 --> 00:03:54,470
has to be on the same indentation level
with try, they are the same block, so
73
00:03:54,470 --> 00:04:02,090
except if there is an exception. What you
want to do is print out: "You are
75
00:04:02,090 --> 00:04:07,540
dividing by zero", or
"is this meaningless". So let me try
77
00:04:16,299 --> 00:04:26,380
this now and you get: "Zero division is
meaningless" and none, oh sorry I meant
79
00:04:26,380 --> 00:04:34,420
to do here return, not print.
I explained you why you get none there so you
81
00:04:34,420 --> 00:04:42,940
got none as an output because what
the function did was its tried to do
83
00:04:42,940 --> 00:04:48,160
this expression but it got an error
so it ignores this line, it doesn't
85
00:04:48,160 --> 00:04:54,880
execute it and then it goes to the
next line. So this line is executed when
87
00:04:54,880 --> 00:05:01,540
this is not executed and so it printed
out: "Zero division is meaningless", but it
89
00:05:01,540 --> 00:05:06,010
also printed out none because when you
execute a function that doesn't have a
91
00:05:06,010 --> 00:05:10,450
return statement such as in this case we
had the print statement here, the print
93
00:05:10,450 --> 00:05:15,820
function not the return statement, in that
case if the function is not returning
95
00:05:15,820 --> 00:05:23,440
anything it can have a print function or
other function as well, but if it
97
00:05:23,440 --> 00:05:29,229
doesn't have a return statement
then it will print out none, so basically
99
00:05:29,229 --> 00:05:33,520
it executes the other procedures such as
the print statement, it prints out the
101
00:05:33,520 --> 00:05:37,690
statement but if it doesn't have
a return statement it doesn't, it prints
103
00:05:37,690 --> 00:05:43,030
out none. Anyway save this now, execute
again and in this case you get only this
105
00:05:43,030 --> 00:05:48,729
zero division is meaningless printed
out there. So basically if you had other
107
00:05:48,729 --> 00:05:57,280
lines of code here like that, those would
be executed as well and that happens by
109
00:05:57,280 --> 00:06:03,160
using the try and except block who which
make sure that the code flow continues.
111
00:06:03,160 --> 00:06:11,050
So while the important thing to note is
that it is advisable to actually here use
113
00:06:11,050 --> 00:06:18,120
the exact name of the error that you
want to accept, so
115
00:06:19,350 --> 00:06:27,340
Zero Division Error and as you see these
got turned into blue which means that
117
00:06:27,340 --> 00:06:33,370
this is actually a Python keyword, is
recognized by Python and in that case if
119
00:06:33,370 --> 00:06:41,560
you exact you this you get the same
output actually like that or if you pass
121
00:06:41,560 --> 00:06:46,650
a number there that it is possible to
get a division from, you'll get that.
123
00:06:46,650 --> 00:06:53,020
Sorry about the backslash. I typed that
by mistake on my terminal. Execute
125
00:06:53,020 --> 00:06:56,650
again so you get this. And so what I'm
trying to say about the zero division
127
00:06:56,650 --> 00:07:01,090
error is that you know sometimes you
might get other types of errors in your
129
00:07:01,090 --> 00:07:07,930
code, other types of exceptions.
Let's say you have a name error there
131
00:07:07,930 --> 00:07:12,370
and you are saying that except all
errors when you don't pass this you
133
00:07:12,370 --> 00:07:17,919
you're basically including all types of
errors there and that is a bit dangerous for your
135
00:07:17,919 --> 00:07:22,960
code because you know you might have
problems that you don't notice and
137
00:07:22,960 --> 00:07:27,789
basically you are ignoring that, but you
shouldn't. So you should be specific
139
00:07:27,789 --> 00:07:32,830
there, explicit like that. If there are
other types of errors such as a name error,
141
00:07:32,830 --> 00:07:38,349
that name error will crash your
program, so you know what's going on.
143
00:07:38,349 --> 00:07:42,760
You need to be specific.
If you want to handle that error too, then
145
00:07:42,760 --> 00:07:47,380
you can add another exception here.
Except name error for instance. Yeah,
147
00:07:47,380 --> 00:07:52,389
that's about handling errors in Python.
I hope this makes sense! I hope I explained
149
00:07:52,389 --> 00:07:58,330
this well! And yeah, this is about errors.
I'll talk to you in the next lectures.
151
00:07:58,330 --> 00:08:00,000
See you!
-
7:00:42
NellieBean
7 hours ago🔴 LIVE - trying some COD maybe Pals later
23.3K -
1:47:46
SpartakusLIVE
5 hours agoThe Master RIZZLER has entered the building, the 95% REJOICE
16.4K -
29:53
MYLUNCHBREAK CHANNEL PAGE
1 day agoOff Limits to the Public - Pt 1
72K104 -
16:03
Tundra Tactical
7 hours ago $10.44 earnedNew Age Gun Fudds
89.5K14 -
8:22
Russell Brand
12 hours agoThey want this to happen
175K342 -
2:06:43
Jewels Jones Live ®
1 day ago2025 STARTS WITH A BANG! | A Political Rendezvous - Ep. 104
96.2K35 -
4:20:41
Viss
12 hours ago🔴LIVE - PUBG Duo Dominance Viss w/ Spartakus
72.3K9 -
10:15:14
MDGgamin
15 hours ago🔴LIVE-Escape From Tarkov - 1st Saturday of 2025!!!! - #RumbleTakeover
59.9K2 -
3:54:19
SpartakusLIVE
11 hours agoPUBG Duos w/ Viss || Tactical Strategy & HARDCORE Gameplay
72.1K1 -
5:54:54
FRENCHY4185
12 hours agoFRENCHY'S BIRTHDAY BASH !!! THE BIG 40 !!!
81.6K3